If’s & Switches
A Little Review
Let’s review, in our last lesson, we learned a couple commands- from adafruit_circuitplayground.express import cpx︎we’ll basically copy this for most of our code, so we don’t need to memorize it, but know that we need it for when we type anything that starts with cpx
- cpx.pixels.fill()︎allows us to write to each of the 10 neopixels on our board at one time. The value in the parenthesis will be three numbers from 0 - 255, which will determine the brightness and color, so cpx.pixels.fill((0,255,0)) will give us green. Note the double parenthesis.
- import time︎this allows us to use anything that starts with time, namely time.sleep(), speaking of...
- time.sleep()︎this allows us to stop everything for a fixed amount of time. The number in the parenthesis will be seconds. So 1 is 1 second while 0.5 will give us half a second and so on.
- while True: ︎is what we use to create a continuous loop. Anything indented after this line will be part of the continuous loop.
Conditional Logic
In order to do more than just turn lights on and off, we’ll need what’s called conditional logic. Conditional logic, allows us to respond to....conditions...logically. That’s not helpful though right? More fundamentally we want to see if certain things (the conditions) are True or False (the logic) so that we can change how the program runs. The point is the latter part; changing how a program runs. We need a way to change the program dynamically. If’s and Else’s
Today we’ll be learning about if statements. They allow us to check...if something is True or False. If it is True, then we’ll do something. One application we can use for this is to check if the buttons or switches are depressed on our Circuit Playground.
Here’s our new code︎
Here’s what our code will do:
[video of switch making pixels go on and off]
Let’s walk through the code
- cpx.switch︎ gives us access to the slide switch on our board. If the USB plug is facing up, the switch to the left will evaulate to True and to the right will be False.
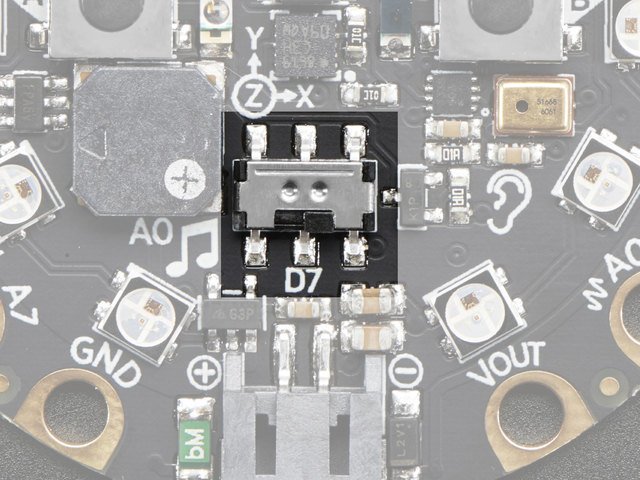
- if cpx.switch == True:︎is our if statement. We are saying “if the value of cpx.switch is True, then do all the code that is indented after it.” The switch will be True when it is to the left, when it is True we want it turn on all the neopixels.
Note the == this is because, we are checking a value rather than setting a value, whenever we want to check a condition, usually via an if statement we need to use ==. - else:︎is what happens if our if statement is False; in this case if the switch is to the right. As an exercise, this code example without the else statement to see what happens.
Elif’s and Some More Switches
So this code will check whether one thing is True or False, what if we want, or need to check other things? Let’s see.[what the code does]
So here’s what our new code does
- cpx.button_a and cpx.button_b︎ These give us access to the two buttons on our board (they are physically labeled A and B). They will be True if they are being pressed, and False while released
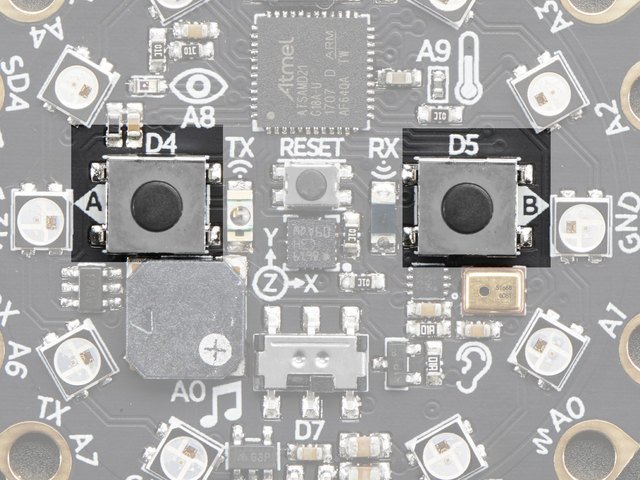
- Elif ︎ This is “else if” We want to check another condition. In our example we wanto check the slide switch, button A and button B. Our code checks each of them in sequence, it says “check if the slide switch is to the left ︎ check if button A is pressed ︎ check if button B is pressed ︎ if none of those are True, turn everything off.”
Print Some Shtuff
It’s pretty important to understand conditional logic, so let’s add some code in order understand what’s happening, we’ll also do print() statements which will become super helpful as we learn to code in python
print() will print to our console. If you press the button that says “Serial” in our editor, you’ll see our print statements appearing and changing as we press our buttons or slide the switch.
Note we’ve added a time.sleep() statement here just so the words fly by a little slowlyer
print() will print to our console. If you press the button that says “Serial” in our editor, you’ll see our print statements appearing and changing as we press our buttons or slide the switch.
Note we’ve added a time.sleep() statement here just so the words fly by a little slowlyer
Stop! Play a Little Here
It’s pretty critical to get this stuff. Change this last example around a bit based on what you don’t understand. Do the following:- Change all the elif’s to if’s to see how it changes the functionality, the main thing you’ll want to check here is what happens if you press both buttons simultaneiously.