Fall 2022 ︎︎︎ SUNY Purchase ︎︎︎ (DES3090) Interactive & Experience Design
CPX Warm up #1
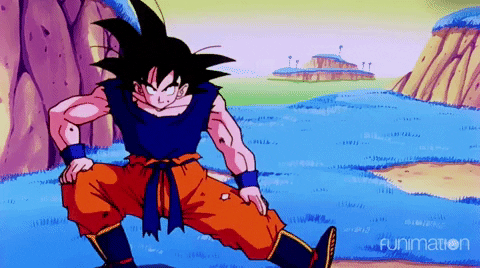
(you may need to refresh to see the code)
Background
As we talked about previously we’ll be doing these so:-
the programming knowledge doesn’t go away
-
you have some more stuff that will supplement your grade
-
you internalize the basic principles of programming
The skeleton
Here’s the basic skeleton of code we’ll be using.︎︎︎You might want to just save this in a separate file that you copy/paste from, or try to type it out from scratch such that you just memorize it. All it does is import the code we need specific to the CPX board, and the Python code we need to do stuff with time.
“while True:” simply gives us our loop so that the code continues executing (try the next example without this to see what happens). Everything indented after it is part of our loop.
Note that in Mu, if you don’t do anything with “import.time” you will receive a warning that you have no time related code. This is fine, Mu is just trying to help you optimize your code.
Light it up
All we’re adding is one line: cp.pixels.fill((255,255,255)). You should remember seeing this before. If you’re wondering the terminology around this stuff, when we have a bunch of periods like this we are accessing an object. The subsequent periods are members of that object which may be other objects, functions or variables.
- “cp” means we want to do something with the Circuit Playground that the import statement allows us to acess to.
- “pixels” means we want to do something with the neopixels; in this case all of them.
- “fill()” is a function that allows us assign a color to all the neopixels; ie “fill” them with a color.
- “(255, 255, 255)” is the color that we want to fill the neopixels with, we need three numbers in a range from 0 - 255. (0, 0, 0) will be the same as turning them off, and the values go in order of R, G, B. When values have parentheses around them like this; it is called a tuple. This is not disssimlar to a list or an array you may have seen in other languages (which also exist in Python). It just allows us to have one variable with multiple values for R, G, and B separately.
Here’s how to light up each neopixel separately︎︎︎
For whatever reason, when you light up a neopixel individually you need to use this syntax. “pixels” is an array of 10 neopixels which we can access individually with the brackets. This is how arrays and tuples are accessed in many programm
ing languages. Note that the first neopixel is 0 rather than 1. So we go from 0 through 9. Setting that equal to a tuple with a color, we are able to light up an individual neopixel.
Here’s how to use time︎︎︎
“time.sleep()” just allows us to do nothing for a little bit. This is supposed to be in seconds, so we use a small value here to wait 1/5 of a second. We turn the lights on and then off and they appear to flash.
Exercises
(make sure that you upload each code example to the respective area in the spreadsheet. Create a separate .py for each exercise. Please make the files are named clearly, they need at least, your name, the week/warmup #, and the exercise number so something like “SantiagoBenjamin__WU01__E01.py” would be something I might name my files, but you’re not obligated to use this exact syntax.)- Make all neopixels light up red.
- Make all neopixels light up green.
- Make all neopixels light up blue.
- Make neopixels #7 and #1 light up a color that looks like purple or pink. (note that this is not a trick question that I am assuming the first neopixel is #0 and the last one would be #9)
- Write a piece of code that does the following:
︎ Make all the neopixels light up a color of your choice, wait a little bit,
︎ turn all the lights off, wait a little bit,
︎ light up all the neopixels another arbitrary color, wait,
︎ then turn them off again, and then wait. - The code below doesn’t work properly, make it work correctly (note that all the neopixels should light up in sequence). It should look like the below image.Add a comment (# in front of the line to write a human language comment) about what line was causing the issue and another comment identifying what you did to fix it.︎︎︎
- Write code that does the following:
︎Make neopixel #0 light up a color that looks like yellow or orange,
︎turn neopixel #0 off,
︎light up neopixel #4 a color that looks like sky blue,
︎ turn neopixel #4 off,
︎and then light up neopixel #9 a color that looks like pink,
︎then turn neopixel #9 off.
(Wait a small amount between each step.) - Make each neopixel light up in sequence (0 -9) any color you want and then turn off in reverse sequence. It should look like the below example.
(note that this is also not a trick question, you are welcome to use for loops, but not expected to)
︎Back to Interactive & Experience Design