Fall 2021 ︎︎︎ SUNY Purchase ︎︎︎ (DES3090) Interactive & Experience Design
CPX Warm up #2
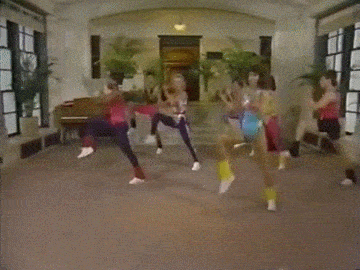
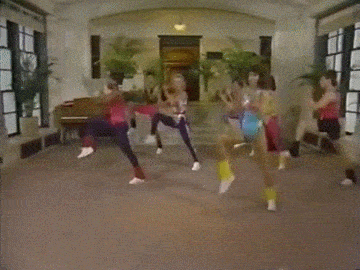
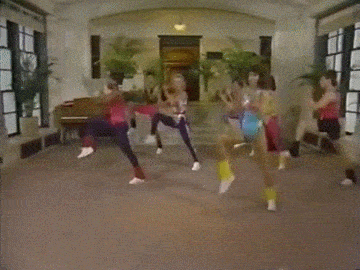
(you may need to refresh to see the code)
Introduction
So last week, we talked simply about lights. This week we’ll talk about for loops. For loops are important in that they allow you to automate some of the tedium that you may have encountered last week. We want to do this because, typing the same text multiple times is:- not “fun”
- introduces the possibility of human error
- is not expandable
Review
Here are all the commands that we went over last week:- cp.pixels.fill((255, 255, 255)) ︎︎︎ lighting up all neopixels
- cp.pixels[0] = (255, 255, 255) ︎︎︎lighting up a single neopixel
- time.sleep(0.2) ︎︎︎ waiting, in seconds
What is a for loop for?
A for loop is one of the ways we can do the same thing multiple times; in the case of the CPX it will be to light up lights, or to control the animation of lights. Here’s a metaphor, it isn’t perfect, but imagine this. You are a king (assume all the kingdoms in this universe do not see the role of king as gender-based), and a neighboring king (your political enemy) has sent over a bushel of apples as a “gift.” You suspect at least one of them might be poisoned. You contract me; a lowly jester; to test the apples for poison. In my loyalty to your kingdom, I agree. How might you command me to check the bushel of apples for poison? Just think about the actual words you would say.
Okay. Now pretend I, the jester, am the computer and you have to tell me (in Python) to perform the same task. You might say something like this︎︎︎
Assuming we have these objects/variables to access (bushel and the king’s decrees) this is one way it might look. This is to say that you would not say… “pick up that apple, bite it to check for poison, set it aside, pick up the next apple, check it for poison by biting it ,okay cool, put it aside”….etc. At some point in theory we’d get the picture.
The little brain in our CPX needs to be told (at least for now) how many times to check a given thing, or when to stop.
The simplest for loops we could use with the neopixels would look like this︎︎︎
Our for loop starts with “for p in range(10):” this means, we will go through a range of values from 0 - 10 (when you only put one number, the first number in the range is assumed to be zero) and we will use the variable “p” for the number
that is changing (0 - 10) each time we go through the loop. Everything that is indented after the colon is the code we will loop, or iterate, through.
- p will start at 0
- the indented code gets executed
- 1 will get added to p
- p will be checked against the top of our range; in this case 10.
- if p is less than 10, the code will execute again
- if p is 10, the code will move on
Note that p is a variable, meaning it can be anything. People often use i for a for loop. You could use the word “neopixel” to be super clear about what you’re modifying. I used “p” for pixel as it is just less typing.
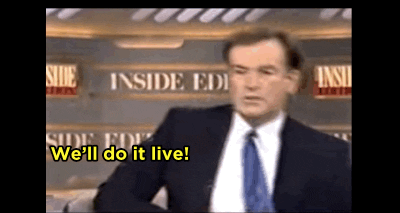
Doing it “live”
If you want to see this happen “live” we can slow our loop down and put a bunch of print statements. Run the following code with the serial console open to see the print statements happening alongside your CPX︎︎︎In the parentheses before the colon we can have, up to three numbers. When we have two numbers we are defining the beginning and end of the range︎︎︎
In this example we are targeting neopixels 0 - 4 to make them red, then neopixels 5 - 9 to make them blue. Note that I didn’t use “p” here for the variable the for loop uses.
Here’s an example using all three possible parameters︎︎︎
When we use three numbers, the numbers determine the start and end of the range, and the third number determines how big each step is. If we only use one or two parameters, the computer assumes we mean to increment (gradually increase) by 1. We can use this to move by 2’s, 4’s 387234’s. We can also use it to move backwards with negative numbers. Here’s the last exercise from last week but with for loops. Note how few lines of code we are using to express the same thing︎︎︎
Not just for targeting individual neopixels
We can use the variable in the for loop to target whatever we want; any number that is changing, or any “group” (array, tuple) of content. Here we are using the variable in the for loop to change the brightness/color of the pixel.︎︎︎Exercises
(make sure that you upload each code example to the respective area in the spreadsheet. Create a separate .py for each exercise. Please make the files are named clearly, they need at least, your name, the week/warmup #, and the exercise number so something like “SantiagoBenjamin__WU2__E01.py” would be something I might name my files, but you’re not obligated to use this exact syntax.)- Make all neopixels “glow” (smoothly turn on and then off) an arbitrary color of your choice.
- Turn each even pixel on and off in sequence (zero is even), then turn each odd pixel on and off in sequence. It should look like this:
- Make all the neopixels “glow” blue and then “glow” red.
- The following code will break, fix it so that it will loop continually.︎︎︎
- Make each neopixel quickly “glow” white in sequence. It should look like this:
(This is the closest to a “trick” question but just combines what is already discussed above)
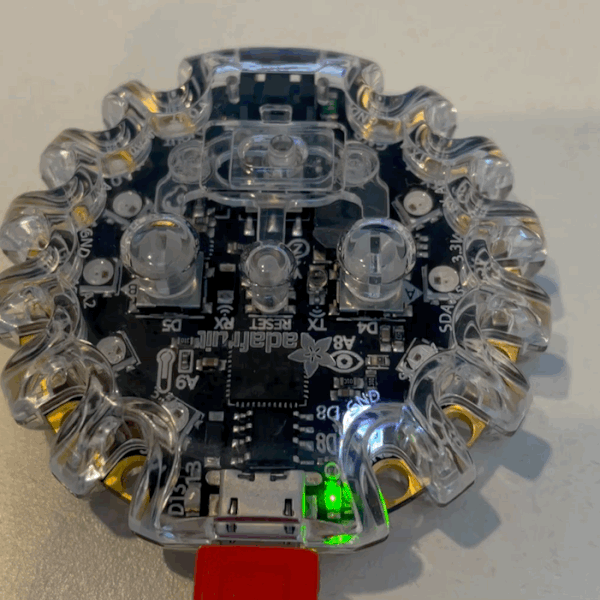
(note this involves nothing new except adding or subtracting or multiplying. This also will result in extra credit if you get it close to “correct”)
Make the CPX do the following, light up each pixel in sequence such that starts “pink” (255, 10, 255) and then gradually becomes “teal” (0, 255, 10) and then turns off. Then lights each pixel in sequence but starts “teal” and becomes “pink”. It should look like this:
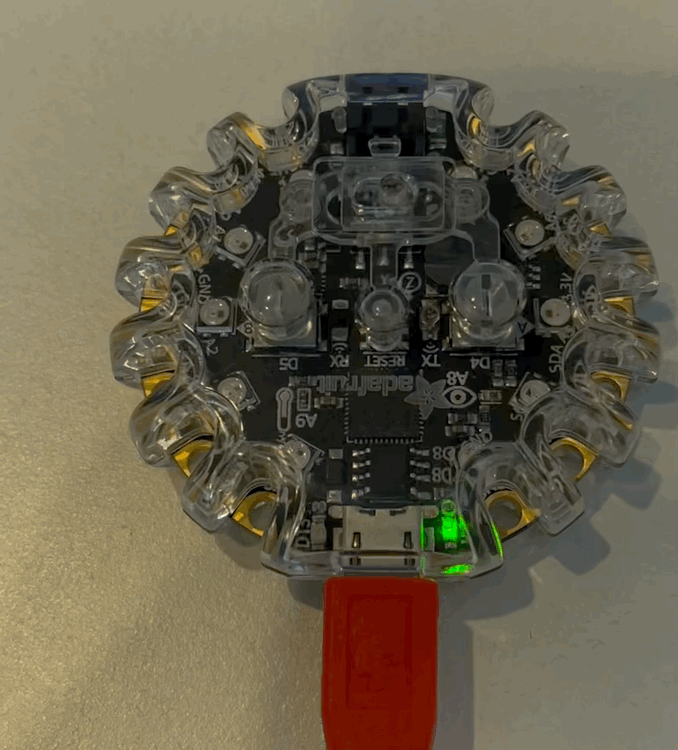
︎Back to Interactive & Experience Design