Fall 2022 ︎︎︎ SUNY Purchase ︎︎︎ (DES3090) Interactive & Experience Design
CPX Warm up #3
what if?
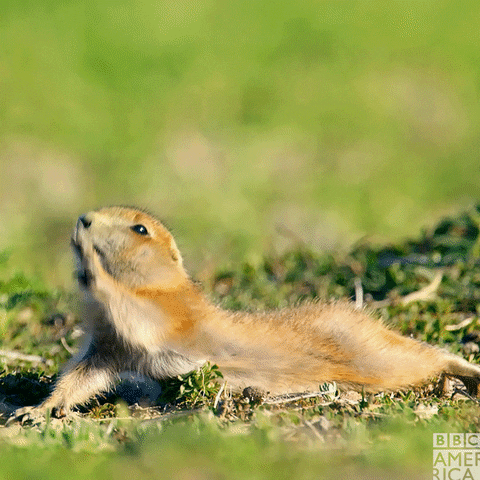
(you may need to refresh to see the code)
What (is an) if (statement)?
Up until now, we’ve only made a program that simply executes a list of steps. We’ve found ways to make things easier, but as you are programming you may want to do things like respond to input (buttons for example), or change surroundings in the environment. Below is a simple example showing how we can light up the neopixels if the “a” button is depressed.If statement introduction︎︎︎
In nerd terms, this is what we call “conditional logic.” That is to say, we check a condition and then continue based upon the result of that checking process. One common way to do that is with what is called an “if statement.” We essentially say “if [something] is True or False...we do [something else]” it is our job to know what those some...things are.
The previous statement is so common, that many programming languages allow you to simplify such a statement, assuming thatyou want to know if it is true.
If statement without Boolean︎︎︎
What else?
In our previous examples, when we release the button, nothing happens. The light does not turn off. In order to evaluate, whether we are not pressing the button we could check whether the button is “True” and then check if it is “False.” However, this is again, such a common situation, that there is something called an else statement that allows use to see what happens if the opposite of our initial check happens. To simplify, here’s the same statement above, allowing us to check if the button is “True” and “False.”
If statment with else︎︎︎
...and then?
Sometimes we want to check multiple things. Think of a realistic situation...if we are at the train station on time, and at the proper track. The immediate situation here is to check the buttons and the sliding switch. To bring multiple checks in one if statement we join them with “and” this means they must both be True for the subsequent statement to execute. If statement with elif (with "and")︎︎︎
This is a zone where you do have some options. Another thing you can do is “nest” statements or place them within each other. In the below example, rather that place multiple statements “anded” together, we have multiple ifs nested within eachother. Neither is wrong or right and are ultimately a matter of preference. I like the “and” as it uses more natural language and is easier to think through. Again it is up to you.
Nested if statements︎︎︎
...or what?
The other logical option you have is “or.” When you use “or” rather than “and” it means that if any of the statements are true the subsequent statement will execute. In the below example we move through a for loop and then any time we encounter an even number we will turn the pixels on one color, otherwise we’ll use a different color.If statements without buttons (and "or")︎︎︎
Exercises
(make sure that you upload each code example to the respective area in the spreadsheet. Create a separate .py for each exercise. Please make the files are named clearly, they need at least, your name, the week/warmup #, and the exercise number so something like “SantiagoBenjamin__WU3__E01.py” would be something I might name my files, but you’re not obligated to use this exact syntax.)- Make it such that button A makes all the pixels turn blue (0, 0, 255) when the button is pressed and then turn off when the button is released.
- Make it such that button B will make all the pixels turn green (0, 255, 0) when the button is released and appear off when the button is pressed.
- Make it such that button A will make neopixels 0 - 4 turn blue, and button B will make neopixels 5 - 9 turn red (they will be off if no button is pressed)
- Make code such that, pressing button A will make all the neopixels blue, pressing button B will make all the neopixels red, and pressing both A and B will make all the neopixels pink.
- Make it such that every other neopixel will be either green or red. That is to say neopixel 0 is green, neopixel 1 is red, etc.
- Start with your example from exercise #5 (every other neopixel is green or red), but amend it such that the switch will control whether the even pixels (0, 2, etc.) are either blue or green.
In other words, if the switch is “off”/False neopixels 0, 2, 4, 6, 8 are green, and if the switch is “on”/True neopixels neopixels 0, 2, 4, 6, 8 are blue.
- Start with your answers from Warm-up #2 for Exercises #3 and #5. Make it such that the switch being “off” will show the sequence for Exercise #3 and the switch being “on” will show the sequence for Exercise #5.
- (Challenge/extra credit!) Redo Exercise #5 above (every other pixel is red or green) except using the modulo operator.
︎Back to Interactive & Experience Design